How to run AB tests in React applications
As React has become one of the most popular frameworks for building user interfaces, some challenges have emerged. Most traditional AB testing platforms work by manipulating the DOM after the page is loaded, which can be a problem depending on how your React application runs.
If you're a developer or a product manager, you've probably struggled with AB testing implementations. This post explores various approaches to this challenge and their pros and cons.
Traditionally, there are basically three ways to implement AB tests on React applications: using React state and conditional rendering, using feature flags, or using a third-party AB testing platform. As an alternative, you could also try a component CMS, such as Croct, using these templates.
Before diving into the technical approaches, it's crucial to understand the foundational principles of AB testing.
-
Randomization
The first step in any AB test is to ensure that users are randomly assigned to different test groups. This ensures that the test is unbiased and that the results are statistically meaningful.
-
Control vs. test group
Every AB test must have a control group (usually the original component version) and one or more test groups (variations). The goal is to compare performance between the control and test groups.
-
Performance considerations
Rendering multiple versions of the same component can cause performance issues in your React app. You must take care to ensure that AB testing doesn't negatively impact the user experience.
With Croct, you can segment your audiences, create AB tests in minutes and improve conversions without or hurting website performance.
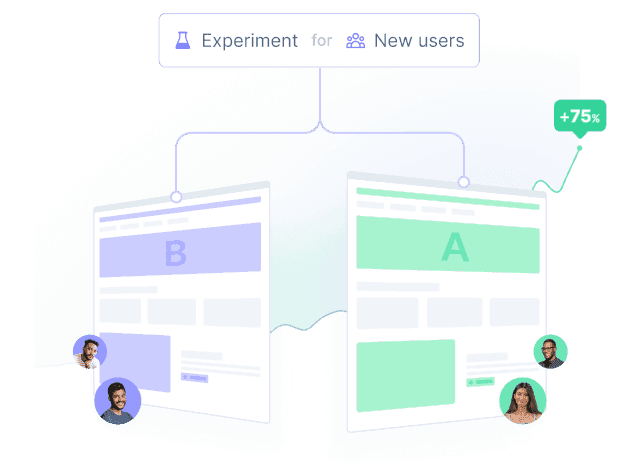
How to implement AB tests in React applications
There are several ways to implement AB tests in React, each with advantages and challenges.
Using React state and conditional rendering
A quick way to run an AB test in React applications is by leveraging state and conditional rendering. This approach allows you to create multiple component versions and toggle between them based on a random assignment.
In the following example, a simple AB test dynamically changes the text of a CTA button based on a random value.
const ABTesting = () => {const [variant, setVariant] = useState(Math.random() > 0.5 ? 'A' : 'B');return (<button>{variant === 'A' ? 'Get Started Now' : 'Join Us Today'}</button>);};
Pros:
- Minimal setup with few external dependencies.
- Great for quick, single-variable tests on a small scale.
Cons:
- Handling randomization and states without a dedicated tool can become complex.
- Users may see different versions across sessions or devices, leading to inconsistent experiences.
- It is unsuitable for complex tests involving multiple segments, user-specific experiences, or analytics integration.
Using feature flags
Feature flags are a more robust way to manage AB testing. They allow you to control the visibility of features and changes dynamically. Libraries like LaunchDarkly and GrowthBook provide powerful solutions for feature flagging.
Pros:
- It allows gradual rollouts, meaning you can test with small user groups and gradually increase exposure.
- Real-time toggling lets you turn features on or off without redeploying code.
Cons:
- Requires integrating an external feature flagging service.
- It can increase the complexity of managing experiments.
Using a third-party AB testing platform
Dedicated AB testing platforms, like VWO and Optimizely, integrate with React applications. These platforms offer sophisticated tools for managing user segments, tracking experiments, and analyzing results.
Pros:
- Comprehensive experiment management with built-in analytics and tracking.
- There is no need to manage randomization or user assignment manually.
Cons:
- Integration can sometimes be complex, especially for platforms without a React SDK.
- Some platforms may introduce performance lags or flickering.
Using Croct for AB testing in React applications
Croct takes AB testing further by enabling segmented AB testing and dynamic content personalization within React applications. Growth and product teams love using it for the developer experience and the performance focus.
Pros:
- You can dynamically tailor content to individual users based on real-time behavior and attributes, such as geolocation.
- It supports both client-side and server-side testing, reducing flicker and performance issues typical of client-side-only solutions.
- It is designed for teams to run experiments efficiently without needing deep technical expertise. An intuitive dashboard provides actionable insights.
- You can start small and scale AB tests as your team grows, integrating seamlessly with your existing stack.
Cons:
- Croct requires an initial setup for full integration, though it offers an SDK with extensive support for modern frameworks like React.
Make everyone happy with the tools the growth team loves: dynamic content, personalization, AB testing, and analytics.
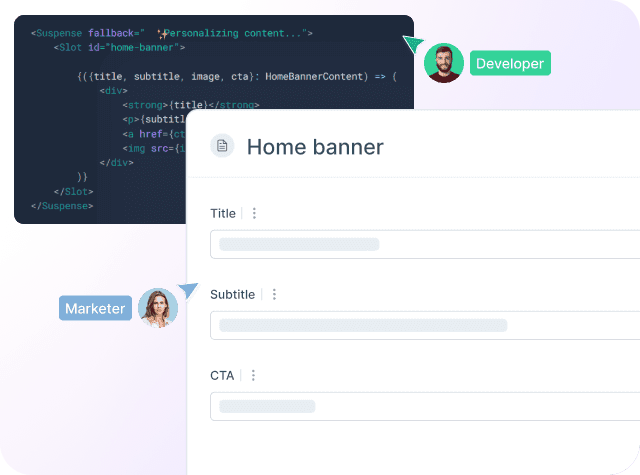
Server-side vs. client-side AB testing
Client-side experiments are more accessible to set up and quicker to implement, making them ideal for testing visual changes that don't require backend logic. The most common challenges arise when tests run after the page loads since it causes users to briefly see one version before it switches to the test variant (we call it a flicker effect). Also, it may introduce performance issues when rendering multiple variants.
Server-side AB testing, on the other hand, doesn't cause flicker since the decision is made before the page renders. It offers greater flexibility for testing backend logic or complex user flows but requires more infrastructure and development effort than client-side testing if you're not using Croct. Handling data collection and analytics
Tracking metrics is critical to understanding the success of your AB tests. In React, you can use hooks or event handlers to capture user interactions and send this data to your analytics platform.
You can integrate with tools like Google Analytics, Mixpanel, Amplitude, or even Croct built-in analytics to monitor and assess the results of your AB tests. Croct, in particular, allows you to run AB testing without any other third-party tool, which is valuable for growth and product teams looking to iterate quickly and keep the costs low.
Conclusion
AB testing is essential for improving conversion rates and user experiences. React offers multiple ways to implement AB tests, from simple conditional rendering to feature flagging and third-party platforms. While each approach has its pros and cons, Croct stands out for its ability to deliver real-time personalization, AB testing solutions, and ease of integration with modern development workflows.
With the right tools and strategies, you can continuously optimize your React applications, driving better business outcomes.